- A potentiometer is basic mechanical device that provides a varying amount of resistance, when its shaft is moved.
- By allowing voltage to pass through potentiometer into an analog input, you can be able to measure the resistance generated by potentiometer as an analog value
Components Required
- Arduino Board
- 10k ohm Potentiometer
- Wires
Procedure
- Connect one of the outer pins in the potentiometer to the ground.
- Connect the other outer pin to 5 volts
- Connect the middle pin of the potentiometer to the analog pin A0.
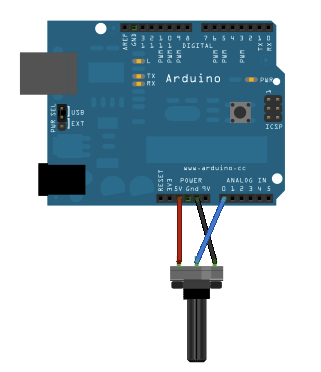
-
Open the Arduino Editor on your computer.
-
In the setup function, type the following:
- In the loop function, declare a variable to store resistance value
int readValue = analogRead(A0);
- On the same loop function print the value to serial monitor like so:
1
2
|
Serial.println(readValue)
|
Full source Code
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
|
// the setup routine runs once when you press reset:
void setup() {
// initialize serial communication at 9600 bits per second:
Serial.begin(9600);
}
// the loop routine runs over and over again forever:
void loop() {
// read the input on analog pin 0:
int sensorValue = analogRead(A0);
// print out the value you read:
Serial.println(sensorValue);
delay(1); // delay in between reads for stability
}
|