Leds are tiny powerful lights that are used in various applications. In these tutorial we shall discuss how to light a blinking led - a hello world program of arduino.
Components Required
- Breadboard
- Arduino Mega 2560(any board you have can work. This tutorial will used Arduino Mega 2560)
- Led
- 330 ohm Resistor
- 2 jumper wired
Procedure
- Set up your connection as shown in the followinf image.
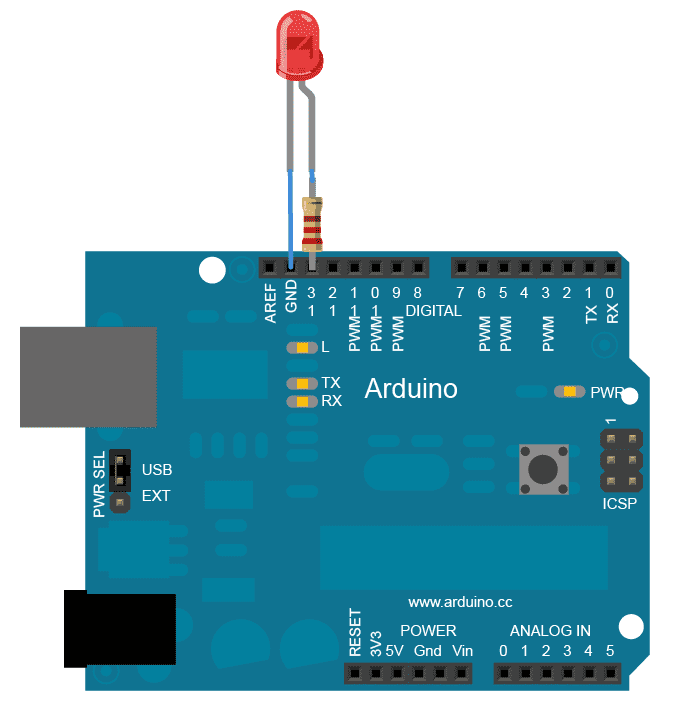
-
Connect your Arduino Board to your Computer
-
Start Arduino IDE
-
Once the Editor is open, Begin by writing the following code in the setup function:
1
2
|
pinMode(LED_BUILTIN, OUTPUT);
|
- In the
loop()
function write:
1
2
3
4
5
6
7
8
|
digitalWrite(LED_BUILTIN, HIGH);
delay(1000)//delay for 1 second
digitalWrite(LED_BUILTIN, LOW); // turn the LED off by making the voltage LOW
delay(1000); // wait for a second
|
Full source Code
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
|
// the setup function runs once when you press reset or power the board
void setup() {
// initialize digital pin LED_BUILTIN as an output.
pinMode(LED_BUILTIN, OUTPUT);
}
// the loop function runs over and over again forever
void loop() {
digitalWrite(LED_BUILTIN, HIGH); // turn the LED on (HIGH is the voltage level)
delay(1000); // wait for a second
digitalWrite(LED_BUILTIN, LOW); // turn the LED off by making the voltage LOW
delay(1000); // wait for a second
}
|